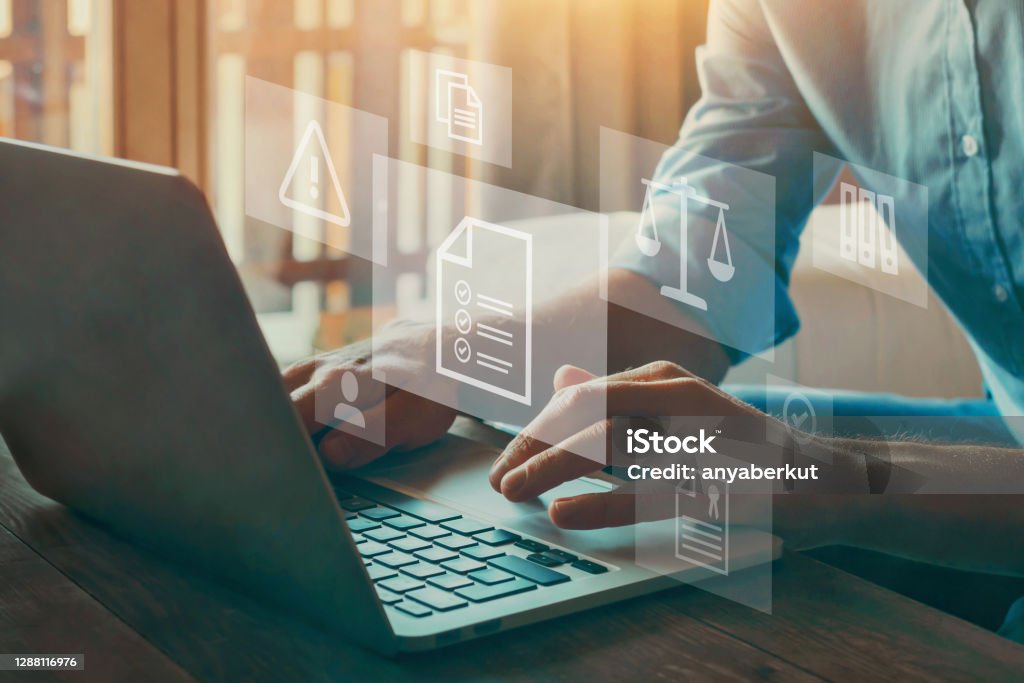
Mybatis-Plus使用(三)条件查询及代码生成器
1. 条件查询器
- 可以添加多个条件查询
@SpringBootTest
public class WrapperTest {
@Autowired
private UserMapper userMapper;
@Test
void test1() {
// 查询name不为空,邮箱不为空,年龄大于等于12
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper
.isNotNull("name")
.isNotNull("email")
.ge("age", 12);
userMapper.selectList(wrapper).forEach(System.out::println);
}
// 条件查询
@Test
void test2() {
// 查询名字等于 levnli
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.eq("name", "levnli");
System.out.println(userMapper.selectOne(wrapper));
}
// 区间查询
@Test
void test3() {
// 查询年龄在20 ~ 30之间
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.between("age", 20, 30);
userMapper.selectList(wrapper).forEach(System.out::println);
}
// 模糊查询
@Test
void test4() {
// 查询年龄在20 ~ 30之间
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper
.notLike("name", "l")
.likeRight("email", "t");
userMapper.selectList(wrapper).forEach(System.out::println);
}
// 嵌套查询
@Test
void test5() {
// 查询年龄在20 ~ 30之间
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.inSql("id","select id from user where id > 3");
userMapper.selectList(wrapper).forEach(System.out::println);
}
}
2. 代码自动生成器
- 减少开发过程中的手动操作
- 加快开发速度
- 导入依赖
<!--导入swagger-->
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<!--模板引擎-->
<dependency>
<groupId>org.apache.velocity</groupId>
<artifactId>velocity-engine-core</artifactId>
<version>2.2</version>
</dependency>
<!--代码生成器-->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-generator</artifactId>
<version>3.0.5</version>
</dependency>
- 编写生成器配置
public class Generator {
public static void main(String[] args) {
// 需要构建一个代码生成器对象
AutoGenerator mpg = new AutoGenerator();
// 1.全局配置
GlobalConfig gc = new GlobalConfig();
String projectPath = System.getProperty("user.dir");
// 生成路径
gc.setOutputDir(projectPath+"/src/main/java");
// 作者
gc.setAuthor("lihao");
// 是否打开文件夹
gc.setOpen(false);
// 是否覆盖之前的
gc.setFileOverride(false);
// 去Service的I前缀
gc.setServiceName("%sService");
// 主键类型
gc.setIdType(IdType.ID_WORKER);
// 日期类型
gc.setDateType(DateType.ONLY_DATE);
// swagger页面
gc.setSwagger2(true);
// 装入生成器
mpg.setGlobalConfig(gc);
// 2.数据源配置
DataSourceConfig dsc = new DataSourceConfig();
// 地址
dsc.setUrl("jdbc:mysql://localhost:3306/mybatis_plus?useUnicode=true&characterEncoding=utf8&zeroDateTimeBehavior=convertToNull&useSSL=true&serverTimezone=GMT%2B8");
// 驱动
dsc.setDriverName("com.mysql.cj.jdbc.Driver");
// 账号
dsc.setUsername("root");
// 密码
dsc.setPassword("root");
// 类型
dsc.setDbType(DbType.MYSQL);
// 放入构造器
mpg.setDataSource(dsc);
// 3.包的配置
PackageConfig pc = new PackageConfig();
// 模块名
pc.setModuleName("blog");
// 存放代码路径
pc.setParent("com.example");
// 实体文件夹名
pc.setEntity("entity");
// mapper文件夹
pc.setMapper("mapper");
// controller文件夹
pc.setController("controller");
// 放入包配置
mpg.setPackageInfo(pc);
// 4.策略配置
StrategyConfig strategy = new StrategyConfig();
// 映射表名
strategy.setInclude("user");
// 名字下划线转驼峰
strategy.setNaming(NamingStrategy.underline_to_camel);
// 列名下划线转驼峰
strategy.setColumnNaming(NamingStrategy.underline_to_camel);
// 父类实体,没有就用设置
strategy.setSuperEntityClass("");
// 实体引入Lombok
strategy.setEntityLombokModel(true);
// RestController类型
strategy.setRestControllerStyle(true);
// 逻辑删除字段
strategy.setLogicDeleteFieldName("deleted");
// 自动填充设置
TableFill gmtCreate = new TableFill("gmt_create", FieldFill.INSERT);
TableFill gmtModified = new TableFill("gmt_modified", FieldFill.INSERT_UPDATE);
// 装入自动填充
ArrayList<TableFill> tableFills = new ArrayList<>();
tableFills.add(gmtCreate);
tableFills.add(gmtModified);
// 装入策略
strategy.setTableFillList(tableFills);
// 乐观锁字段名
strategy.setVersionFieldName("version");
// 开启驼峰命名
strategy.setRestControllerStyle(true);
// 链接请求添加下划线命名
strategy.setControllerMappingHyphenStyle(true);
mpg.setStrategy(strategy);
// 执行
mpg.execute();
}
}
3. 学习内容总结
- mybatis-plus是在之前的基础之上增加而来,内置了简单的CRUD方法,当使用多表联查的时候,也可以自己创建xml文件编写复杂的SQL语句
- 感谢你赐予我前进的力量
赞赏者名单
因为你们的支持让我意识到写文章的价值🙏
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 木子李
评论
匿名评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果