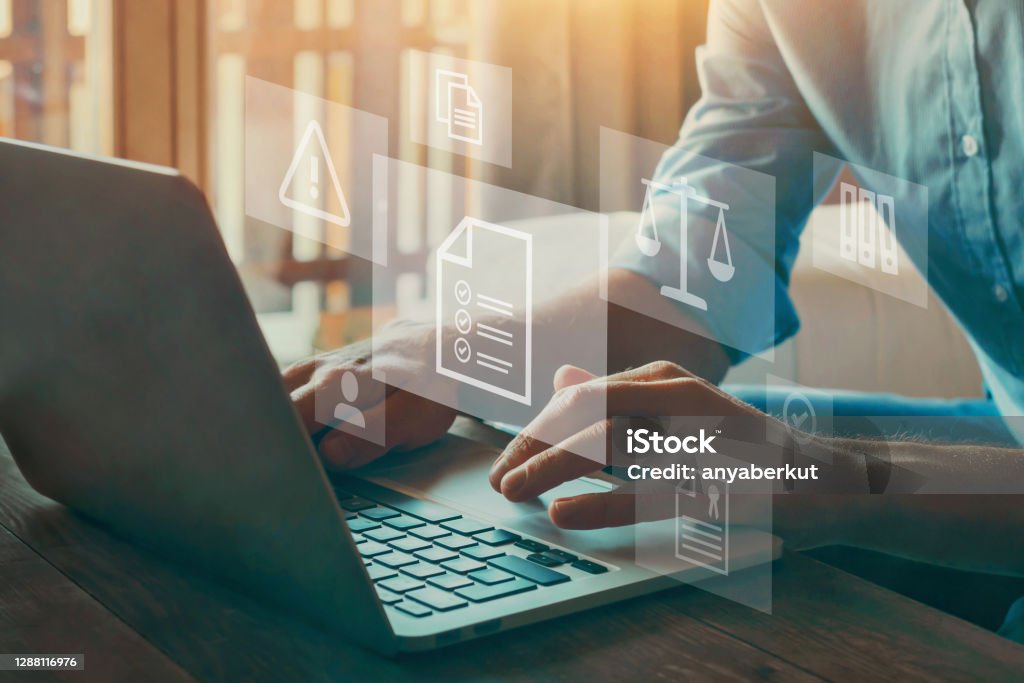
JUC高并发编程
JUC高并发编程
1、什么是JUC
java.util工具包、包、类
业务:普通的线程代码 Thread
Runnable 没有返回值、效率相比于 Callable 相对较低!
2、线程和进程
线程、进程,如果不能使用一句话说出来的技术,不扎实!
进程:一个运行在内存中的程序集合
一个进程往往可以包含多个线程,至少包含一个!
java默认有几个线程?2个 mian、GC(垃圾回收)
线程:开了一个进程Typora,写字,自动保存(线程负责)
对于java而言:Thread、Rnnable、Callable
java真的可以开启线程吗?
通过native调用本地方法,调用底层的C++开启,无法直接操作硬件
并发、并行
并发(多线程操作同一个资源)
- CPU一核,模拟出来多条线程,快速交替
并行(多个人一起行走)
- CPU多核,多个线程可以同时执行;线程池
public class Test1 {
public static void main(String[] args) {
// 获取cpu的核数
// CPU 密集型、IO密集型
System.out.println(Runtime.getRuntime().availableProcessors());
}
}
并发编程的本质:充分利用CPU的资源
所有的公司都很看重!
线程有几个状态
public enum State {
// 创建
NEW,
// 运行
RUNNABLE,
// 阻塞
BLOCKED,
// 等待,死等
WAITING,
// 超时等待
TIMED_WAITING,
// 终止
TERMINATED;
}
wait/sleep 区别
1、来自不同的类
wait=>Object
sleep=>Thread
2、关于锁的释放
wait会释放锁,sleep不会释放
3、使用的范围不同
wait 必须在同步代码块中
sleep 可以在任何地方睡
4、是否需要捕获异常
wait不需要
sleep必须要捕获异常
3、Lock锁(重点)
传统synchronized
// 基本的卖票例子
/**
* 真正的多线程开发,公司中的开发,降低耦合性
* 线程就是一个单独的资源类,没有任何的附属的操作!
* 1、属性、方法
*/
public class SaleTicketDemo01 {
public static void main(String[] args) {
// 并发:多线程操作同一个资源类,把资源类丢入线程
Ticket ticket = new Ticket();
// @FunctionalInterface 函数式接口
new Thread(() -> {
for (int i = 0; i < 40; i++) {
ticket.sale();
}
}, "A").start();
new Thread(() -> {
for (int i = 0; i < 40; i++) {
ticket.sale();
}
}, "B").start();
new Thread(() -> {
for (int i = 0; i < 40; i++) {
ticket.sale();
}
}, "C").start();
}
}
// 资源OOP,解耦
class Ticket {
// 属性、方法
private int number = 30;
// 卖票的方式
public synchronized void sale() {
if (number > 0) {
System.out.println(Thread.currentThread().getName() + "卖出了" + (number--) + "票,剩余:" + number);
}
}
}
Lock
公平锁:十分公平,可以先来后到
非公平锁:可以插队(默认)
package demo01;
// 基本的卖票例子
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
/**
* 真正的多线程开发,公司中的开发,降低耦合性
* 线程就是一个单独的资源类,没有任何的附属的操作!
* 1、属性、方法
*/
public class SaleTicketDemo02 {
public static void main(String[] args) {
// 并发:多线程操作同一个资源类,把资源类丢入线程
Ticket2 ticket = new Ticket2();
// @FunctionalInterface 函数式接口, jdk1.8 lambda表达式 (参数)->{ 代码 }
new Thread(() -> { for (int i = 0; i < 40; i++) ticket.sale(); }, "A").start();
new Thread(() -> { for (int i = 0; i < 40; i++) ticket.sale(); }, "B").start();
new Thread(() -> { for (int i = 0; i < 40; i++) ticket.sale(); }, "C").start();
}
}
// Lock
/**
* new ReentrantLock
* lock.lock()
* finally => lock.unlock
*/
class Ticket2 {
// 属性、方法
private int number = 30;
Lock lock = new ReentrantLock();
// 卖票的方式
public void sale() {
// 加锁
lock.lock();
try {
if (number > 0) {
System.out.println(Thread.currentThread().getName() + "卖出了" + (number--) + "票,剩余:" + number);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 解锁
lock.unlock();
}
}
}
synchronized 和 lock 区别
1、synchronized 内置的java关键字,lock是一个java类
2、synchronized 无法判断获取锁的状态,lock 可以判断是否获取到了锁
3、synchronized 会自动释放锁,lock 必须要手动释放锁,如果不释放锁,死锁
4、synchronized 线程1(获得锁,阻塞),线程2(等待,死等);lock 锁就不一定会等待下去;
5、synchronized 可重入锁,不可以中断,非公平;lock 可重入锁,可以判断锁,非公平(默认),可以自己设置;
6、synchronized 适合锁少量的代码同步问题,lock 适合锁大量的同步代码!
锁是什么?如何判断锁的是谁?
4、生产者和消费者问题
面试:单例模式、排序算法、生产者和消费者、死锁
synchronized版
package pc;
/**
* 线程之间的通信问题,生产者和消费者问题 等待唤醒,通知唤醒
* 线程交替执行 A B 操作同一个变量 num = 0
* A num+1
* B num-1
*/
public class A {
public static void main(String[] args) {
Data data = new Data();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.increment();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "A").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.decrement();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "B").start();
}
}
class Data {
// 数字 资源类
private int number = 0;
// +1
public synchronized void increment() throws InterruptedException {
if (number != 0) {
// 等待
this.wait();
}
number++;
System.out.println(Thread.currentThread().getName() + "=>" + number);
// 通知其他线程,我+1完毕
this.notifyAll();
}
// -1
public synchronized void decrement() throws InterruptedException {
if (number == 0) {
// 等待
this.wait();
}
number--;
System.out.println(Thread.currentThread().getName() + "=>" + number);
// 通知其他线程,我-1完毕
this.notifyAll();
}
}
问题存在,A、B、C、D 4个线程还安全么?
if改为while判断
package pc;
/**
* 线程之间的通信问题,生产者和消费者问题 等待唤醒,通知唤醒
* 线程交替执行 A B 操作同一个变量 num = 0
* A num+1
* B num-1
*/
public class A {
public static void main(String[] args) {
Data data = new Data();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.increment();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "A").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.decrement();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "B").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.increment();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "C").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.decrement();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "D").start();
}
}
class Data {
// 数字 资源类
private int number = 0;
// +1
public synchronized void increment() throws InterruptedException {
while (number != 0) {
// 等待
this.wait();
}
number++;
System.out.println(Thread.currentThread().getName() + "=>" + number);
// 通知其他线程,我+1完毕
this.notifyAll();
}
// -1
public synchronized void decrement() throws InterruptedException {
while (number == 0) {
// 等待
this.wait();
}
number--;
System.out.println(Thread.currentThread().getName() + "=>" + number);
// 通知其他线程,我-1完毕
this.notifyAll();
}
}
JUC版的生产者和消费者
代码实现
package pc;
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
/**
* 线程之间的通信问题,生产者和消费者问题 等待唤醒,通知唤醒
* 线程交替执行 A B 操作同一个变量 num = 0
* A num+1
* B num-1
*/
public class B {
public static void main(String[] args) {
Data2 data = new Data2();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.increment();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "A").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.decrement();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "B").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.increment();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "C").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.decrement();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "D").start();
}
}
class Data2 {
// 数字 资源类
private int number = 0;
Lock lock = new ReentrantLock();
Condition condition = lock.newCondition();
// +1
public void increment() throws InterruptedException {
lock.lock();
try {
while (number != 0) {
// 等待
condition.await();
}
number++;
System.out.println(Thread.currentThread().getName() + "=>" + number);
// 通知其他线程,我+1完毕
condition.signalAll();
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
// -1
public void decrement() throws InterruptedException {
lock.lock();
try {
while (number == 0) {
// 等待
condition.await();
}
number--;
System.out.println(Thread.currentThread().getName() + "=>" + number);
// 通知其他线程,我-1完毕
condition.signalAll();
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
}
任何一个新的技术,绝对不是紧紧知识覆盖了原来的技术,优势和补充!
Condition 精准通知和唤醒线程
package pc;
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
/**
* A 执行完调用 B
* B 执行完调用 C
* C 执行完调用 A
*/
public class C {
public static void main(String[] args) {
Data3 data = new Data3();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
data.printA();
}
}, "A").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
data.printB();
}
}, "B").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
data.printC();
}
}, "C").start();
}
}
class Data3 {
private final Lock lock = new ReentrantLock();
private final Condition condition1 = lock.newCondition();
private final Condition condition2 = lock.newCondition();
private final Condition condition3 = lock.newCondition();
private int number = 1;
public void printA() {
lock.lock();
try {
// 业务,判断->执行->通知
while (number != 1) {
// 等待
condition1.await();
}
System.out.println(Thread.currentThread().getName() + "=>>AAAAAA");
// 唤醒指定的线程,B
number = 2;
condition2.signal();
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
public void printB() {
lock.lock();
try {
// 业务,判断->执行->通知
while (number != 2) {
// 等待
condition2.await();
}
System.out.println(Thread.currentThread().getName() + "=>>BBBBBB");
// 唤醒指定的线程,C
number = 3;
condition3.signal();
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
public void printC() {
lock.lock();
try {
// 业务,判断->执行->通知
while (number != 3) {
// 等待
condition3.await();
}
System.out.println(Thread.currentThread().getName() + "=>>CCCCCC");
// 唤醒指定的线程,C
number = 1;
condition1.signal();
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
}
5、8锁现象
如何判断锁的是谁!永远的知道什么锁,锁的到底是谁!
对象、Class
深刻理解锁
package lock8;
import java.util.concurrent.TimeUnit;
/**
* 8锁,关于锁的8个问题
* 1、标准情况下,两个线程先打印 发短信?还是打电话?
* 2、sendSms延迟4秒,两个线程先打印 发短信还是打电话?
*/
public class Test1 {
public static void main(String[] args) {
Phone phone = new Phone();
// 锁的存在
new Thread(() -> {
phone.sendSms();
try {
TimeUnit.SECONDS.sleep(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
}, "A").start();
new Thread(() -> {
phone.call();
}, "B").start();
}
}
class Phone {
// synchronized 锁的对象是方法的调用者
// 两个方法用的是同一个锁,谁先拿到谁执行
public synchronized void sendSms() {
try {
TimeUnit.SECONDS.sleep(4);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("发短信");
}
public synchronized void call() {
System.out.println("打电话");
}
}
package lock8;
import java.util.concurrent.TimeUnit;
/**
* 8锁,关于锁的8个问题
* 3、增加了一个普通方法后,发短信还是hello?
* 4、两个对象,两个同步方法,发短信还是打电话?
*/
public class Test2 {
public static void main(String[] args) {
// 两个对象
Phone2 phone = new Phone2();
Phone2 phone2 = new Phone2();
// 锁的存在
new Thread(() -> {
phone.sendSms();
try {
TimeUnit.SECONDS.sleep(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
}, "A").start();
new Thread(() -> {
phone2.call();
}, "B").start();
}
}
class Phone2 {
// synchronized 锁的对象是方法的调用者
// 两个方法用的是同一个锁,谁先拿到谁执行
public synchronized void sendSms() {
try {
TimeUnit.SECONDS.sleep(4);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("发短信");
}
public synchronized void call() {
System.out.println("打电话");
}
// 没有锁,不是同步方法,不受影响
public void hello() {
System.out.println("hello");
}
}
package lock8;
import java.util.concurrent.TimeUnit;
/**
* 8锁,关于锁的8个问题
* 5、增加两个静态的同步方法,只有一个对象,先打印发短信?打电话?
* 6、两个对象!增加两个静态的同步方法,只有一个对象,先打印发短信?打电话?
*/
public class Test3 {
public static void main(String[] args) {
// 两个对象的Class类模板只有一个,static,锁的是Class
Phone3 phone = new Phone3();
Phone3 phone2 = new Phone3();
// 锁的存在
new Thread(() -> {
phone.sendSms();
try {
TimeUnit.SECONDS.sleep(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
}, "A").start();
new Thread(() -> {
phone2.call();
}, "B").start();
}
}
// Phone3唯一的一个 class对象
class Phone3 {
// synchronized 锁的对象是方法的调用者
// static 静态方法
// 类一加载就有了!Class模板
public static synchronized void sendSms() {
try {
TimeUnit.SECONDS.sleep(4);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("发短信");
}
public static synchronized void call() {
System.out.println("打电话");
}
}
package lock8;
import java.util.concurrent.TimeUnit;
/**
* 8锁,关于锁的8个问题
* 7、一个静态的同步方法,一个普通静态同步方法,一个对象
* 8、一个静态的同步方法,一个普通静态同步方法,两个对象
*/
public class Test4 {
public static void main(String[] args) {
// 两个对象的Class类模板只有一个,static,锁的是Class
Phone4 phone = new Phone4();
Phone4 phone2 = new Phone4();
// 锁的存在
new Thread(() -> {
phone.sendSms();
try {
TimeUnit.SECONDS.sleep(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
}, "A").start();
new Thread(() -> {
phone2.call();
}, "B").start();
}
}
// Phone3唯一的一个 class对象
class Phone4 {
// 静态同步方法 锁的是class类模板
public static synchronized void sendSms() {
try {
TimeUnit.SECONDS.sleep(4);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("发短信");
}
// 普通同步方法 锁的调用者
public synchronized void call() {
System.out.println("打电话");
}
}
小结
new this 具体的一个手机
static Class唯一的一个模板
6、集合类不安全
List不安全
package unsafe;
import java.util.*;
import java.util.concurrent.CopyOnWriteArrayList;
/**
* java.util.ConcurrentModificationException 并发修改异常!
*/
public class ListTest {
public static void main(String[] args) {
// 并发下 ArrayList 不安全
/**
* 解决方案:
* 1、List<String> list = new Vector<>();
* 2、List<String> list = Collections.synchronizedList(new ArrayList<>());
* 3、List<String> list = new CopyOnWriteArrayList<>();
*/
// 写入时复制 COW 计算机程序设计领域的一种优化策略
// 多个线程调用的时候,list,读取的时候,是固定的,写入的时候(覆盖)
// 在写入的时候避免覆盖,造成数据问题
// 读写分离 MyCat
// CopyOnWriteArrayList 比 Vector 厉害在哪里?
// Vector使用的是synchronized,效率较低,CopyOnWriteArrayList使用的是lock锁
List<String> list = new CopyOnWriteArrayList<>();
for (int i = 0; i < 10; i++) {
new Thread(()->{
list.add(UUID.randomUUID().toString().substring(0,5));
System.out.println(list);
},String.valueOf(i)).start();
}
}
}
Set不安全
package unsafe;
import java.util.Collections;
import java.util.HashSet;
import java.util.Set;
import java.util.UUID;
import java.util.concurrent.CopyOnWriteArraySet;
/**
* 同理可证:java.util.ConcurrentModificationException
*/
public class SetTest {
public static void main(String[] args) {
// Set<String> set = new HashSet<>();
// Set<String> set = Collections.synchronizedSet(new HashSet<>());
Set<String> set = new CopyOnWriteArraySet<>();
for (int i = 1; i <= 30; i++) {
new Thread(()->{
set.add(UUID.randomUUID().toString().substring(0,5));
System.out.println(set);
},String.valueOf(i)).start();
}
}
}
hashSet底层是什么?
public HashSet() {
map = new HashMap<>();
}
// add set 本质就是map的key是无法重复的!
public boolean add(E e) {
return map.put(e, PRESENT)==null;
}
// 不变的值
private static final Object PRESENT = new Object();
Map不安全
package unsafe;
import java.util.HashMap;
import java.util.Map;
import java.util.UUID;
import java.util.concurrent.ConcurrentHashMap;
/**
* 同理可证:java.util.ConcurrentModificationException
*/
public class MapTest {
public static void main(String[] args) {
// map 是这样用的么? 不是,工作中不用HashMap
// 默认等价于什么? new HashMap<16,0.75>();
// Map<String, String> map = new HashMap<>();
Map<String, String> map = new ConcurrentHashMap<>();
for (int i = 1; i <= 30; i++) {
new Thread(() -> {
map.put(Thread.currentThread().getName(),UUID.randomUUID().toString().substring(0, 5));
System.out.println(map);
}, String.valueOf(i)).start();
}
}
}
7、Callable(简单)
1、可以有返回值
2、可以抛出异常
3、方法不同,run()、call()
代码测试
package callable;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.FutureTask;
public class CallableTest {
public static void main(String[] args) throws ExecutionException, InterruptedException {
// futureTask做适配器,
FutureTask<String> futureTask = new FutureTask<>(new MyThread());
new Thread(futureTask,"A").start();
new Thread(futureTask,"B").start();
// 打印返回结果,get方法可能会产生阻塞,获取返回值通常放在最后
// 或者使用异步通信处理
System.out.println(futureTask.get());
}
}
class MyThread implements Callable<String> {
@Override
public String call() throws Exception {
System.out.println("call()");
return "ok";
}
}
细节:
1、有缓存
2、结果可能需要等待,会阻塞
8、常用的辅助类(必会)
8.1、CountDownLatch
加法计数器
package add;
import java.util.concurrent.CountDownLatch;
public class CountDownLatchDemo {
public static void main(String[] args) throws InterruptedException {
// 总数是6,再必须要执行任务的时候,再使用
CountDownLatch countDownLatch = new CountDownLatch(6);
for(int i = 0;i < 6;i ++){
new Thread(()->{
System.out.println(Thread.currentThread().getName()+"Go out");
// 数量-1
countDownLatch.countDown();
},String.valueOf(i)).start();
}
// 等待计数器归零,再向下执行
countDownLatch.await();
System.out.println("Close door");
}
}
原理:
==countDownLatch.countDown();==数量-1
==countDownLatch.await();==等待计数器归零,然后再向下执行
每次有线程调用countDown()数量-1,假设计数器变为0,countDownLatch.await()就会被唤醒,继续执行
8.2、CyclicBarrier
减法计数器
package add;
import java.util.concurrent.BrokenBarrierException;
import java.util.concurrent.CyclicBarrier;
public class CyclicBarrierDemo {
public static void main(String[] args) {
CyclicBarrier cyclicBarrier = new CyclicBarrier(7,()->{
System.out.println("召唤神龙成功");
});
for(int i = 1;i <= 7;i ++){
final int temp = i;
new Thread(()->{
System.out.println(Thread.currentThread().getName()+"收集了"+temp+"个龙珠");
// 等待
try {
cyclicBarrier.await();
} catch (InterruptedException e) {
e.printStackTrace();
} catch (BrokenBarrierException e) {
e.printStackTrace();
}
}).start();
}
}
}
8.3、Semaphore
中间信号量
==semaphore.acquire();==获得,假设如果已经满了,等待,等待被释放为止
==semaphore.release();==释放,会将当前的信号量释放+1,然后唤醒等待的线程!
作用:多个共享资源互斥使用!并发限流,控制最大的线程数!
package add;
import java.util.concurrent.Semaphore;
import java.util.concurrent.TimeUnit;
public class SemaphoreDemo {
public static void main(String[] args) {
// 线程数量:
Semaphore semaphore = new Semaphore(3);
for(int i = 1;i <= 6;i ++){
new Thread(()->{
// acquire() 得到
try {
semaphore.acquire();
System.out.println(Thread.currentThread().getName()+"抢到车位");
TimeUnit.SECONDS.sleep(2);
System.out.println(Thread.currentThread().getName()+"离开车位");
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
// release() 释放
semaphore.release();
}
},String .valueOf(i)).start();
}
}
}
9、读写锁
ReadWriteLock
package readWriteLock;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.locks.ReadWriteLock;
import java.util.concurrent.locks.ReentrantReadWriteLock;
/**
* 独占锁(写锁) 一次只能被一个线程占有
* 共享锁(读锁) 多个线程可以同时占有
* ReadWriteLock 读写锁
* 读-读 可以共存
* 读-写 不可共存
* 写-写 不可共存
*/
public class ReadWriteLockDemo {
public static void main(String[] args) {
//MyCache myCache = new MyCache();
MyCacheLock myCache = new MyCacheLock();
// 写入
for (int i = 0; i < 5; i++) {
final int temp = i;
new Thread(()->{
myCache.put(String.valueOf(temp),String.valueOf(temp));
},String.valueOf(i)).start();
}
// 读取
for (int i = 0; i < 5; i++) {
final int temp = i;
new Thread(()->{
myCache.get(String.valueOf(temp));
},String.valueOf(i)).start();
}
}
}
/**
* 加锁自定义缓存
*/
class MyCacheLock {
private volatile Map<String, Object> map = new HashMap<>();
// 读写锁,更加细粒度的控制
private ReadWriteLock lock = new ReentrantReadWriteLock();
// 存,写入的时候,只希望同时只有一个线程写
public void put(String key,Object value){
lock.writeLock().lock();
try {
System.out.println(Thread.currentThread().getName()+"写入"+key);
map.put(key, value);
System.out.println(Thread.currentThread().getName()+"写入OK");
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.writeLock().unlock();
}
}
// 取,读,所有人都可以读
public void get(String key){
lock.readLock().lock();
try {
System.out.println(Thread.currentThread().getName()+"读取"+key);
Object o = map.get(key);
System.out.println(Thread.currentThread().getName()+"读取OK");
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.readLock().unlock();
}
}
}
/**
* 自定义缓存
*/
class MyCache {
private volatile Map<String, Object> map = new HashMap<>();
// 存,写
public void put(String key,Object value){
System.out.println(Thread.currentThread().getName()+"写入"+key);
map.put(key, value);
System.out.println(Thread.currentThread().getName()+"写入OK");
}
// 取,读
public void get(String key){
System.out.println(Thread.currentThread().getName()+"读取"+key);
Object o = map.get(key);
System.out.println(Thread.currentThread().getName()+"读取OK");
}
}
10、阻塞队列
阻塞队列:
BlockingQueue不是新的东西
什么情况下会使用阻塞队列:多线程并发处理,线程池
学会使用队列
添加、移除
四组API
方式 | 抛出异常 | 不会抛异常,有返回值 | 阻塞等待 | 超时等待 |
---|---|---|---|---|
添加 | add() | offer() | put() | offer(E,T,G) |
移除 | remove() | poll() | take() | poll(T,G) |
查看队首元素 | element() | peek() | - | - |
1、抛出异常
2、不会抛出异常
3、阻塞等待
4、超时等待
package blockingQueue;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.TimeUnit;
public class Test {
public static void main(String[] args) throws InterruptedException {
//test1();
//test2();
//test3();
test4();
}
/**
* 抛出异常
*/
public static void test1(){
// 队列的大小
ArrayBlockingQueue blockingQueue = new ArrayBlockingQueue<>(3);
System.out.println(blockingQueue.add("a"));
System.out.println(blockingQueue.add("b"));
System.out.println(blockingQueue.add("c"));
// IllegalStateException:队列已满
//System.out.println(blockingQueue.add("c"));
// 查看队首元素
System.out.println(blockingQueue.element());
System.out.println(blockingQueue.remove());
System.out.println(blockingQueue.remove());
System.out.println(blockingQueue.remove());
// NoSuchElementException:空元素
//System.out.println(blockingQueue.remove());
}
/**
* 不抛异常
*/
public static void test2(){
ArrayBlockingQueue blockingQueue = new ArrayBlockingQueue<>(3);
System.out.println(blockingQueue.offer("a"));
System.out.println(blockingQueue.offer("b"));
System.out.println(blockingQueue.offer("c"));
// false 不抛异常
//System.out.println(blockingQueue.offer("d"));
// 查看队首元素
System.out.println(blockingQueue.peek());
System.out.println(blockingQueue.poll());
System.out.println(blockingQueue.poll());
System.out.println(blockingQueue.poll());
// null 无异常
System.out.println(blockingQueue.poll());
}
/**
* 等待,阻塞(死等)
*/
public static void test3() throws InterruptedException {
ArrayBlockingQueue blockingQueue = new ArrayBlockingQueue<>(3);
// 一直阻塞
blockingQueue.put("a");
blockingQueue.put("b");
blockingQueue.put("c");
// 没有位置,死等
//blockingQueue.put("d");
System.out.println(blockingQueue.take());
System.out.println(blockingQueue.take());
System.out.println(blockingQueue.take());
// 没有元素,死等
//System.out.println(blockingQueue.take());
}
/**
* 等待,阻塞(等待超时)
*/
public static void test4() throws InterruptedException {
ArrayBlockingQueue blockingQueue = new ArrayBlockingQueue<>(3);
blockingQueue.offer("a");
blockingQueue.offer("b");
blockingQueue.offer("c");
blockingQueue.offer("d",2, TimeUnit.SECONDS);
System.out.println("==================");
System.out.println(blockingQueue.poll());
System.out.println(blockingQueue.poll());
System.out.println(blockingQueue.poll());
// null 无异常
System.out.println(blockingQueue.poll(2,TimeUnit.SECONDS));
}
}
SynchronousQueue 同步队列
没有容量,
package blockingQueue;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.SynchronousQueue;
import java.util.concurrent.TimeUnit;
/**
* 同步队列
* 与其他的BlockingQueue 不一样, SynchronousQueue 不存储元素
* put 了一个元素,必须从里面先take 取出,否则不能再 put 进去值
*/
public class SynchronousQueueDemo {
public static void main(String[] args) {
BlockingQueue<String> blockingQueue = new SynchronousQueue<>();
new Thread(() -> {
try {
System.out.println(Thread.currentThread().getName() + "put 1");
blockingQueue.put("1");
System.out.println(Thread.currentThread().getName() + "put 2");
blockingQueue.put("2");
System.out.println(Thread.currentThread().getName() + "put 3");
blockingQueue.put("3");
} catch (Exception e) {
e.printStackTrace();
}
}, "T1").start();
new Thread(() -> {
try {
TimeUnit.SECONDS.sleep(3);
System.out.println(Thread.currentThread().getName() + "=" + blockingQueue.take());
TimeUnit.SECONDS.sleep(3);
System.out.println(Thread.currentThread().getName() + "=" + blockingQueue.take());
TimeUnit.SECONDS.sleep(3);
System.out.println(Thread.currentThread().getName() + "=" + blockingQueue.take());
} catch (InterruptedException e) {
e.printStackTrace();
}
}, "T2").start();
}
}
11、线程池(重点)
线程池:三大方法、七大参数、四种拒绝策略
池化技术
程序的运行,本质:占用系统的资源!优化资源的使用!=>池化技术
线程池、连接池、内存池、对象池。。。// 创建、销毁。十分浪费资源
池化技术:事先准备好一些资源,有人要用,就来池中取,用完归还
线程池的好处:
1、降低资源的消耗
2、提高响应的速度
3、方便管理
线程复用、可以控制最大并发数、管理线程
线程池:三大方法
package poll;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
/**
* Executors 工具类:3大方法
* 通过创建线程池来调用线程
*/
public class Demo01 {
public static void main(String[] args) {
// 单个线程
//ExecutorService threadPool = Executors.newSingleThreadExecutor();
// 创建一个固定的线程池大小
ExecutorService threadPool = Executors.newFixedThreadPool(5);
// 可伸缩的,遇强则强,遇弱则弱
//ExecutorService threadPool = Executors.newCachedThreadPool();
try {
for (int i = 0; i < 10; i++) {
threadPool.execute(() -> {
System.out.println(Thread.currentThread().getName() + " OK");
});
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 线程池用完,程序结束,关闭线程池
threadPool.shutdown();
}
}
}
七大参数
源码分析
public static ExecutorService newSingleThreadExecutor() {
return new FinalizableDelegatedExecutorService
(new ThreadPoolExecutor(1, 1,
0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>()));
}
public static ExecutorService newFixedThreadPool(int nThreads) {
return new ThreadPoolExecutor(nThreads, nThreads,
0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>());
}
public static ExecutorService newCachedThreadPool() {
return new ThreadPoolExecutor(0, Integer.MAX_VALUE,
60L, TimeUnit.SECONDS,
new SynchronousQueue<Runnable>());
}
本质:ThreadPoolExecutor()
public ThreadPoolExecutor(int corePoolSize, // 核心大小
int maximumPoolSize, // 最大核心大小
long keepAliveTime, // 存活事件
TimeUnit unit, // 超时单位
BlockingQueue<Runnable> workQueue, // 阻塞队列
ThreadFactory threadFactory, // 线程工厂,创建线程,一般不用动
RejectedExecutionHandler handler // 拒绝策略) {
if (corePoolSize < 0 ||
maximumPoolSize <= 0 ||
maximumPoolSize < corePoolSize ||
keepAliveTime < 0)
throw new IllegalArgumentException();
if (workQueue == null || threadFactory == null || handler == null)
throw new NullPointerException();
this.acc = System.getSecurityManager() == null ?
null :
AccessController.getContext();
this.corePoolSize = corePoolSize;
this.maximumPoolSize = maximumPoolSize;
this.workQueue = workQueue;
this.keepAliveTime = unit.toNanos(keepAliveTime);
this.threadFactory = threadFactory;
this.handler = handler;
}
手动创建线程池
package poll;
import java.util.concurrent.*;
public class Demo02 {
public static void main(String[] args) {
ExecutorService threadPool = new ThreadPoolExecutor(
2,
5,
3,
TimeUnit.SECONDS,
new LinkedBlockingQueue<>(3),
Executors.defaultThreadFactory(),
// 超出不处理
//new ThreadPoolExecutor.AbortPolicy());
// 哪来的回哪里
//new ThreadPoolExecutor.CallerRunsPolicy());
// 队列满了不会抛出异常,会丢掉任务
//new ThreadPoolExecutor.DiscardPolicy());
// 队列满了,尝试和最早的竞争,也不会抛出异常
new ThreadPoolExecutor.DiscardOldestPolicy());
try {
// 最大承载:队列长度+最大核心数
for (int i = 0; i < 9; i++) {
threadPool.execute(() -> {
System.out.println(Thread.currentThread().getName() + " OK");
});
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 线程池用完,程序结束,关闭线程池
threadPool.shutdown();
}
}
}
四种拒绝策略
1、超出不处理
2、哪来的回哪去
3、队列满了,尝试和最早的竞争,不抛异常
4、队列满了不会抛异常,丢弃任务
小结和拓展
线程池的最大线程数到底该如何设置
1、CPU密集型,根据CPU核心数定义,可以保证CPU效率最高
Runtime.getRuntime().availableProcessors() 获取CPU核心数
2、IO密集型,判断程序中十分耗IO的线程,通常双倍设置
12、四大函数式接口(重点,必须掌握)
新时代程序员:lambda表达式、链式编程、函数式接口、Stream流式计算
函数式接口:只有一个方法的接口
@FunctionalInterface
public interface Runnable {
public abstract void run();
}
// 超级多FunctionalInterface
// 简化编程模型,在新版本的框架底层大量应用
// foreach(消费者类的函数式接口)
Function函数式接口
package function;
import java.util.function.Function;
/**
* Function 函数型接口, 有一个输入参数,有一个输出
* 只要是函数型接口,可以用 lambda 表达式简化
*/
public class Demo01 {
public static void main(String[] args) {
//Function function = (Function<String, String>) str -> str;
Function function = (str) -> str;
System.out.println(function.apply("abc"));
}
}
断定型接口:有一个输入参数,返回值只能是 布尔值!
package function;
import java.util.function.Predicate;
/**
* 断定型接口:有一个输入参数,返回值只能是 布尔值!
*/
public class Demo02 {
public static void main(String[] args) {
// 判断字符串是否为空
//Predicate<String> predicate = new Predicate<String>() {
// @Override
// public boolean test(String s) {
// return s.isEmpty();
// }
//};
Predicate<String> predicate = (str)->{return str.isEmpty();};
System.out.println(predicate.test(""));
}
}
Consumer 消费型接口
package function;
import java.util.function.Consumer;
/**
* Consumer 消费型接口:只有输入,没有返回值
*/
public class Demo03 {
public static void main(String[] args) {
//Consumer<String> consumer = new Consumer<String>() {
// @Override
// public void accept(String o) {
// System.out.println(o);
// }
//};
Consumer<String> consumer = (str)->{System.out.println(str);};
consumer.accept("abc");
}
}
Supplier 供给型接口
package function;
import java.util.function.Supplier;
/**
* Supplier 供给型接口 没有参数,只有返回值
*/
public class Demo04 {
public static void main(String[] args) {
//Supplier<Integer> supplier = new Supplier<Integer>() {
// @Override
// public Integer get() {
// System.out.println("get()");
// return 1024;
// }
//};
Supplier<Integer> supplier = ()->{return 1024;};
System.out.println(supplier.get());
}
}
13、Stream流式计算
什么是Stream流式计算
大数据:存储 + 计算
存储:集合、MySQL 本质就是存储
计算都应该交给流来操作!
package stream;
public class User {
private Integer id;
private String name;
private Integer age;
public User(Integer id, String name, Integer age) {
this.id = id;
this.name = name;
this.age = age;
}
public User() {
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
@Override
public String toString() {
return "User{" +
"id=" + id +
", name='" + name + '\'' +
", age=" + age +
'}';
}
}
package stream;
import java.util.Arrays;
import java.util.Comparator;
import java.util.List;
/**
* 题目要求:一分钟内完成此题,只能用一行代码实现!
* 现在有5个用户!筛选
* 1、ID 必须是偶数
* 2、年龄必须大于23岁
* 3、用户名转为大写字母
* 4、用户名字母到着排序
* 5、只输出一个用户!
*/
public class Test {
public static void main(String[] args) {
User u1 = new User(1, "a", 21);
User u2 = new User(2, "b", 22);
User u3 = new User(3, "c", 23);
User u4 = new User(4, "d", 24);
User u5 = new User(6, "e", 25);
// 集合就是存储
List<User> list = Arrays.asList(u1, u2, u3, u4, u5);
// 计算交给Stream流
// lambda表达式、链式编程、函数式接口、Stream流式计算
list.stream()
.filter(u -> u.getId() % 2 == 0)
.filter(u -> u.getAge() > 23)
.map(u -> u.getName().toUpperCase())
.sorted(Comparator.reverseOrder())
.limit(1)
.forEach(System.out::println);
}
}
14、ForkJoin(分支合并)
什么是ForkJoin
JDK1.7出现,并行执行任务!提高效率,大数据量!
大数据:Map Reduce (把大任务拆分为小任务)
ForkJoin 特点:工作窃取
这里面维护的都是双端队列
ForkJoin
ForkJoin样例
package forkJoin;
import java.util.concurrent.RecursiveTask;
/**
* 求和计算的任务
* ForkJoin Stream并行流
* 如何使用ForkJoin
* 1、ForkJoinPool
* 2、计算任务 ForkJoin.execute(ForkJoinTask task)
* 3、计算类要继承ForkJoinTask
*/
public class ForkJoinDemo extends RecursiveTask<Long> {
private Long start;
private Long end;
// 临界值
private Long temp = 10000L;
public ForkJoinDemo(Long start, Long end) {
this.start = start;
this.end = end;
}
// 计算方法
@Override
protected Long compute() {
if ((end - start) < temp) {
long sum = 0L;
for (Long i = start; i <= end; i++) {
sum += i;
}
return sum;
} else {
// 中间值
long middle = (start + end) / 2;
ForkJoinDemo task1 = new ForkJoinDemo(start, middle);
// 拆分任务,把任务压入线程队列
task1.fork();
ForkJoinDemo task2 = new ForkJoinDemo(middle + 1, end);
task2.fork();
return task1.join() + task2.join();
}
}
}
测试类
package forkJoin;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.ForkJoinTask;
import java.util.stream.LongStream;
public class Test {
public static void main(String[] args) throws ExecutionException, InterruptedException {
// 耗时单位毫秒
//test1(); // 6177
//test2(); // 2082
test3(); // 198
}
// 普通做法
public static void test1() {
Long sum = 0L;
long start = System.currentTimeMillis();
for (Long i = 1L; i <= 10_0000_0000L; i++) {
sum += i;
}
long end = System.currentTimeMillis();
System.out.println("sum=" + sum + " 时间:" + (end - start));
}
// ForkJoin 拆分计算
public static void test2() throws ExecutionException, InterruptedException {
long start = System.currentTimeMillis();
ForkJoinPool forkJoinPool = new ForkJoinPool();
ForkJoinTask<Long> task = new ForkJoinDemo(0L, 10_0000_0000L);
// 提交任务
ForkJoinTask<Long> submit = forkJoinPool.submit(task);
Long sum = submit.get();
long end = System.currentTimeMillis();
System.out.println("sum=" + sum + " 时间:" + (end - start));
}
// Stream流式计算
public static void test3() {
long start = System.currentTimeMillis();
long sum = LongStream.rangeClosed(0L, 10_0000_0000L).parallel().reduce(0, Long::sum);
long end = System.currentTimeMillis();
System.out.println("sum=" + sum + " 时间:" + (end - start));
}
}
15、异步回调
Future设计的初衷:对将来的某个事件的结果进行建模
package future;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
/**
* 异步调用:CompletableFuture
* 异步执行
* 成功回调
*/
public class Demo01 {
public static void main(String[] args) throws ExecutionException, InterruptedException {
// 发起一个请求,没有返回值的 runAsync 异步回调
//CompletableFuture<Void> completableFuture = CompletableFuture.runAsync(()->{
// try {
// TimeUnit.SECONDS.sleep(2);
// } catch (InterruptedException e) {
// e.printStackTrace();
// }
// System.out.println(Thread.currentThread().getName()+"runAsync=>Void");
//});
//
//System.out.println("1111111111");
//
//// 阻塞获取执行结果
//completableFuture.get();
// 有返回值的异步回调 supplyAsync
CompletableFuture<Integer> completableFuture = CompletableFuture.supplyAsync(() -> {
System.out.println(Thread.currentThread().getName() + "supplyAsync=>Integer");
// 故意制造错误
int i = 10 / 0;
return 1024;
});
System.out.println(completableFuture.whenComplete((t, u) -> {
// 正确返回信息
System.out.println("t=>" + t);
// 错误信息:java.util.concurrent.CompletionException: java.lang.ArithmeticException: / by zero
System.out.println("u=>" + u);
}).exceptionally((e) -> {
System.out.println(e.getMessage());
// 可以获取到错误的返回结果
return 2333;
}).get());
}
}
16、JMM
请你谈谈对Volatile的理解
Volatile是Java虚拟机提供轻量级的同步机制
1、保证可见性
2、==不保证原子性==
3、禁止指令重排
什么是JMM
JMM:java内存模型,不存在的东西,概念!约定!
关于JMM的一些同步的约定:
1、线程解锁前,必须把共享变量==立刻==刷回主存
2、线程加锁前,必须读取主存中的最新值到工作内存中!
3、加锁和解锁是同一把锁
线程分为:工作内存、主内存
内存交互操作有8种,虚拟机实现必须保证每一个操作都是原子的,不可在分的(对于double和long类型的变量来说,load、store、read和write操作在某些平台上允许例外)
- lock (锁定):作用于主内存的变量,把一个变量标识为线程独占状态
- unlock (解锁):作用于主内存的变量,它把一个处于锁定状态的变量释放出来,释放后的变量才可以被其他线程锁定
- read (读取):作用于主内存变量,它把一个变量的值从主内存传输到线程的工作内存中,以便随后的load动作使用
- load (载入):作用于工作内存的变量,它把read操作从主存中变量放入工作内存中
- use (使用):作用于工作内存中的变量,它把工作内存中的变量传输给执行引擎,每当虚拟机遇到一个需要使用到变量的值,就会使用到这个指令
- assign (赋值):作用于工作内存中的变量,它把一个从执行引擎中接受到的值放入工作内存的变量副本中
- store (存储):作用于主内存中的变量,它把一个从工作内存中一个变量的值传送到主内存中,以便后续的write使用
- write (写入):作用于主内存中的变量,它把store操作从工作内存中得到的变量的值放入主内存的变量中
JMM对这八种指令的使用,制定了如下规则:
- 不允许read和load、store和write操作之一单独出现。即使用了read必须load,使用了store必须write
- 不允许线程丢弃他最近的assign操作,即工作变量的数据改变了之后,必须告知主存
- 不允许一个线程将没有assign的数据从工作内存同步回主内存
- 一个新的变量必须在主内存中诞生,不允许工作内存直接使用一个未被初始化的变量。就是怼变量实施use、store操作之前,必须经过assign和load操作
- 一个变量同一时间只有一个线程能对其进行lock。多次lock后,必须执行相同次数的unlock才能解锁
- 如果对一个变量进行lock操作,会清空所有工作内存中此变量的值,在执行引擎使用这个变量前,必须重新load或assign操作初始化变量的值
- 如果一个变量没有被lock,就不能对其进行unlock操作。也不能unlock一个被其他线程锁住的变量
- 对一个变量进行unlock操作之前,必须把此变量同步回主内存
问题:程序不知道主内存的值已经被修改过了
17、Volatile
1、保证可见性
package tvolatile;
import java.util.concurrent.TimeUnit;
public class JMMDemo {
// 如果不加 volatile 就会死循环
// 加 volatile 保证可见性
private volatile static int num = 0;
public static void main(String[] args) {
// 线程1 对主内存的变化不知道
new Thread(()->{
while (num==0){
}
}).start();
try {
TimeUnit.SECONDS.sleep(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
num = 1;
System.out.println(num);
}
}
2、不保证原子性
原子性:不可分割
线程A在执行任务的时候,不能被打扰的,也不能被分割。要么同时成功,要么同时失败
package tvolatile;
/**
* volatile 不保证原子性
*/
public class VDemo02 {
private volatile static int num = 0;
public static void add() {
num++;
}
public static void main(String[] args) {
for (int i = 1; i <= 20; i++) {
new Thread(() -> {
for (int i1 = 0; i1 < 1000; i1++) {
add();
}
}).start();
}
while (Thread.activeCount() > 2) {
Thread.yield();
}
System.out.println(Thread.currentThread().getName() + " " + num);
}
}
如果不加lock和synchronized,怎么样保证原子性
使用java.util.concurrent.atomic原子类解决
package tvolatile;
import java.util.concurrent.atomic.AtomicInteger;
/**
* volatile 不保证原子性
*/
public class VDemo02 {
private volatile static AtomicInteger num = new AtomicInteger();
public static void add() {
// num++;不是一个原子性操作
//AtomicInteger+1操作
num.getAndIncrement();
}
public static void main(String[] args) {
for (int i = 1; i <= 20; i++) {
new Thread(() -> {
for (int i1 = 0; i1 < 1000; i1++) {
add();
}
}).start();
}
while (Thread.activeCount() > 2) {
Thread.yield();
}
System.out.println(Thread.currentThread().getName() + " " + num);
}
}
这些类的底层都直接和操作系统挂钩!在内存中修改值!Unsafe类是一个很特殊的存在!
3、指令重排
什么 指令重排:编写的程序,计算机并不是按照编写的内容去执行
源代码-->编译器优化的重排-->指令并行也可能重排-->内存系统也会重排-->执行
指令重排的前提:处理器在进行指令重排的时候,会考虑:数据之间的依赖性!
可能造成影响的结果: a b x y 这四个值默认都是 0
线程A | 线程B |
---|---|
x=a | y=b |
b=1 | a=2 |
正常结果:x = 0; y = 0; 但是可能由于指令重排
线程A | 线程B |
---|---|
x=a | a=2 |
b=1 | y=b |
指令重排导致的诡异结果:x = 2; y = 1;
volatile 可以避免指令重排
内存屏障,CPU指令。作用:
1、保证特定操作的执行顺序!
2、保证某些变量的内存可见性(利用这些特性volatile实现了可见性)
Volatile 是可以保证可见性,不能保证原子性,由于内存屏障,可以保证避免指令重排的现象
18、彻底玩转单例模式
饿汉式,DCL懒汉式
饿汉式
package single;
/**
* 饿汉式单例
*/
public class Hungry {
private Hungry() {
}
private final static Hungry HUNGRY = new Hungry();
public static Hungry getInstance() {
return HUNGRY;
}
}
DCL 懒汉式
package single;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
/**
* 懒汉式单例
*/
public class LazyMan {
private static boolean flag = false;
private LazyMan() {
synchronized (LazyMan.class) {
if (flag == false) {
flag = true;
} else {
throw new RuntimeException("不要试图使用反射破坏异常");
}
}
System.out.println(Thread.currentThread().getName() + "ok");
}
private volatile static LazyMan lazyMan;
// 双重检测所模式的懒汉式单例 DCL懒汉式
public static LazyMan getInstance() {
// 加锁
if (lazyMan == null) {
synchronized (LazyMan.class) {
if (lazyMan == null) {
/**
* 不是原子性操作
* 1、分配内存空间
* 2、执行构造方法,初始化对象
* 3、把这个对象指向内存空间
* 期望顺序 1、2、3
* 可能顺序 1、3、2 A线程
* B线程进来时,已经指向内存空间,但是没有初始化对象
* 此时得到的lazyMan还没完成初始构造
*
* 解决方法在对象上加入volatile
*/
lazyMan = new LazyMan();
}
}
}
return lazyMan;
}
// 反射
public static void main(String[] args) throws Exception {
//LazyMan instance = LazyMan.getInstance();
Field flag = LazyMan.class.getDeclaredField("flag");
flag.setAccessible(true);
Constructor<LazyMan> declaredConstructor = LazyMan.class.getDeclaredConstructor(null);
declaredConstructor.setAccessible(true);
LazyMan instance = declaredConstructor.newInstance();
flag.set(instance, false);
LazyMan instance2 = declaredConstructor.newInstance();
System.out.println(instance);
System.out.println(instance2);
}
}
静态内部类
package single;
/**
* 静态内部类实现
*/
public class Holder {
private Holder() {
}
public static Holder getInstance() {
return InnerClass.HOLDER;
}
public static class InnerClass {
private static final Holder HOLDER = new Holder();
}
}
单例模式不安全可以通过反射破坏
枚举类型的最终反编译源码
package single;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
/**
* 枚举本身也是一个class
*/
public enum EnumSingle {
INSTANCE;
public EnumSingle getInstance() {
return INSTANCE;
}
}
class Test {
public static void main(String[] args) throws NoSuchMethodException, InvocationTargetException, InstantiationException, IllegalAccessException {
EnumSingle instance1 = EnumSingle.INSTANCE;
Constructor<EnumSingle> declaredConstructor = EnumSingle.class.getDeclaredConstructor(String.class, int.class);
declaredConstructor.setAccessible(true);
EnumSingle instance2 = declaredConstructor.newInstance();
// NoSuchMethodException
System.out.println(instance1);
System.out.println(instance2);
}
}
枚举是有参构造
枚举累的的最终反编译源码:
package single;
public final class EnumSingle extends Enum
{
public static EnumSingle[] values()
{
return (EnumSingle[])$VALUES.clone();
}
public static EnumSingle valueOf(String name)
{
return (EnumSingle)Enum.valueOf(single/EnumSingle, name);
}
private EnumSingle(String s, int i)
{
super(s, i);
}
public EnumSingle getInstance()
{
return INSTANCE;
}
public static final EnumSingle INSTANCE;
private static final EnumSingle $VALUES[];
static
{
INSTANCE = new EnumSingle("INSTANCE", 0);
$VALUES = (new EnumSingle[] {
INSTANCE
});
}
}
19、深入理解CAS
什么是 CAS
为什么要学?大厂要深入研究底层,有所突破!修内功,操作系统,计算机网络原理
package cas;
import java.util.concurrent.atomic.AtomicInteger;
public class CASDemo {
// CAS compareAndSet:比较并交换
public static void main(String[] args) {
AtomicInteger atomicInteger = new AtomicInteger(2020);
// 期望、更新
// 如果期望值达到了,就更新,否则不更新, CAS 是CPU的并发原语
System.out.println(atomicInteger.compareAndSet(2020, 2021));
System.out.println(atomicInteger.get());
atomicInteger.getAndIncrement();
System.out.println(atomicInteger.compareAndSet(2020, 2021));
System.out.println(atomicInteger.get());
}
}
Unsafe 类
java通过Unsafe类调用底层操作内存
CAS:比较当前工作内存中的值和主内存中的值,如果这个值是期望的,那么则执行操作!如果不是就一直循环!
缺点:
1、由于是自旋锁,循环会耗时
2、一次性只能保证一个共享变量的原子性
3、ABA问题
CAS: ABA 问题(狸猫换太子)
package cas;
import java.util.concurrent.atomic.AtomicInteger;
public class CASDemo {
// CAS compareAndSet:比较并交换
public static void main(String[] args) {
AtomicInteger atomicInteger = new AtomicInteger(2020);
// 对于平时写的SQL: 乐观锁!
// 期望、更新
// 如果期望值达到了,就更新,否则不更新, CAS 是CPU的并发原语
//============捣乱线程============
System.out.println(atomicInteger.compareAndSet(2020, 2021));
System.out.println(atomicInteger.get());
System.out.println(atomicInteger.compareAndSet(2021, 2020));
System.out.println(atomicInteger.get());
//============期望线程============
System.out.println(atomicInteger.compareAndSet(2020, 2021));
System.out.println(atomicInteger.get());
}
}
20、原子引用
解决ABA问题,引入原子引用!对应的思想:乐观锁
带版本号的原子操作
package cas;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.atomic.AtomicStampedReference;
public class CASDemo {
// CAS compareAndSet:比较并交换
public static void main(String[] args) {
//AtomicStampedReference 注意,如果泛型是一个包装类,注意对象的应用问题
AtomicStampedReference<Integer> atomicInteger = new AtomicStampedReference<>(1, 1);
new Thread(() -> {
// 获得版本号
int stamp = atomicInteger.getStamp();
System.out.println("a1=>" + stamp);
try {
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(atomicInteger.compareAndSet(1, 2,
atomicInteger.getStamp(), atomicInteger.getStamp() + 1));
System.out.println("a2=>" + atomicInteger.getStamp());
System.out.println(atomicInteger.compareAndSet(2, 1,
atomicInteger.getStamp(), atomicInteger.getStamp() + 1));
System.out.println("a3=>" + atomicInteger.getStamp());
}, "A").start();
// 乐观锁的原理相同
new Thread(() -> {
// 获得版本号
int stamp = atomicInteger.getStamp();
System.out.println("b1=>" + stamp);
try {
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(atomicInteger.compareAndSet(1, 6,
stamp, stamp + 1));
System.out.println("b2=>" + atomicInteger.getStamp());
}, "B").start();
}
}
注意:Integer 使用了对象缓存机制,默认范围是-128~127,推荐使用静态工厂方法valueOf获取对象实力,而不是new,因为valueOf使用缓存,而new 一定会创建新的对象分配新的内存空间
21、各种锁的理解
1、公平锁、非公平锁
公平锁:不能够插队,线程有先来后到
非公平锁:可以插队(默认都是飞公平)
public ReentrantLock() {
sync = new NonfairSync();
}
public ReentrantLock(boolean fair) {
sync = fair ? new FairSync() : new NonfairSync();
}
2、可重入锁
可重入锁(递归锁)
package lock;
/**
* Synchronized
*/
public class Demo01 {
public static void main(String[] args) {
Phone phone = new Phone();
new Thread(() -> {
phone.sms();
}, "A").start();
new Thread(() -> {
phone.sms();
}, "B").start();
}
}
class Phone {
public synchronized void sms() {
System.out.println(Thread.currentThread().getName() + " sms");
call();
}
public synchronized void call() {
System.out.println(Thread.currentThread().getName() + " call");
}
}
package lock;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
/**
* Lock版
*/
public class Demo02 {
public static void main(String[] args) {
Phone2 phone = new Phone2();
new Thread(() -> {
phone.sms();
}, "A").start();
new Thread(() -> {
phone.sms();
}, "B").start();
}
}
class Phone2 {
Lock lock = new ReentrantLock();
public synchronized void sms() {
// 实际两次加锁,
lock.lock();
// lock 锁必须配对,几次加锁,必须几次解锁
try {
System.out.println(Thread.currentThread().getName() + " sms");
call();
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
public synchronized void call() {
lock.lock();
try {
System.out.println(Thread.currentThread().getName() + " call");
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
}
3、自旋锁
package lock;
import java.util.concurrent.atomic.AtomicReference;
/**
* 自旋锁
*/
public class SpinLockDemo {
AtomicReference<Thread> atomicReference = new AtomicReference<>();
// 加锁
public void myLock() {
Thread thread = Thread.currentThread();
System.out.println(Thread.currentThread().getName() + "=> myLock");
while (!atomicReference.compareAndSet(null, thread)) {
}
}
// 解锁
public void myUnLock() {
Thread thread = Thread.currentThread();
System.out.println(Thread.currentThread().getName() + "=> myUnLock");
atomicReference.compareAndSet(thread, null);
}
}
package lock;
import java.util.concurrent.TimeUnit;
public class TestSpinLock {
public static void main(String[] args) {
SpinLockDemo lock = new SpinLockDemo();
new Thread(() -> {
lock.myLock();
try {
TimeUnit.SECONDS.sleep(3);
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.myUnLock();
}
}, "T1").start();
try {
TimeUnit.SECONDS.sleep(1);
} catch (Exception e) {
e.printStackTrace();
}
new Thread(() -> {
lock.myLock();
try {
TimeUnit.SECONDS.sleep(1);
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.myUnLock();
}
}, "T2").start();
}
}
T1先获得锁,正常执行,T2也获得锁,然后T2自旋空转,等T1解锁后,T2才能解锁。
4、死锁
死锁是什么?
死锁的必要条件:
1、互斥条件
2、请求和保持
3、不可抢占
4、循环等待
package lock;
import java.util.concurrent.TimeUnit;
public class DeadLockDemo {
public static void main(String[] args) {
String lockA = "lockA";
String lockB = "lockB";
new Thread(new MyThread(lockA, lockB), "T1").start();
new Thread(new MyThread(lockB, lockA), "T1").start();
}
}
class MyThread implements Runnable {
private String lockA;
private String lockB;
public MyThread(String lockA, String lockB) {
this.lockA = lockA;
this.lockB = lockB;
}
@Override
public void run() {
synchronized (lockA) {
System.out.println(Thread.currentThread().getName() + " lock:" + lockA + "=>get" + lockB);
try {
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
synchronized (lockB) {
System.out.println(Thread.currentThread().getName() + " lock:" + lockB + "=>get" + lockA);
}
}
}
}
解决问题
1、使用 jps - l
定位进程号
2、是jstack 进程号
查看程序信息
排查问题方式:
1、日志
2、堆栈信息
- 感谢你赐予我前进的力量